包围盒
定义包围盒
struct BoundBox { BoundBox();
bool isPointInside(D3DXVECTOR3& p);
D3DXVECTOR3 _min; D3DXVECTOR3 _max; }; BoundBox::BoundBox() { _min.x = -Infinity; _min.y = -Infinity; _min.z = -Infinity;
_max.x = Infinity; _max.y = Infinity; _max.z = Infinity; } bool BoundBox::isPointInside(D3DXVECTOR3& p) { if (p.x >= _min.x && p.y >= _min.y && p.z >= _min.z && p.x <= _max.x && p.y <= _max.y && p.z <= _max.z) { return true; } else { return false; } }
|
声明变量
ID3DXMesh* BoxMesh = 0; const float Infinity = FLT_MAX; BoundBox boundingBox;
|
创建包围盒
加载模型
bool ComputeBoundingBox(ID3DXMesh* mesh, BoundBox* box) { HRESULT hr = 0;
BYTE* v = 0; mesh->LockVertexBuffer(0, (void**)&v); hr = D3DXComputeBoundingBox( (D3DXVECTOR3*)v, mesh->GetNumVertices(), D3DXGetFVFVertexSize(mesh->GetFVF()), &box->_min, &box->_max);
mesh->UnlockVertexBuffer();
if (FAILED(hr)) return false;
return true; } ComputeBoundingBox(Mesh, &boundingBox);
D3DXCreateBox( g_pd3dDevice, boundingBox._max.x - boundingBox._min.x, boundingBox._max.y - boundingBox._min.y, boundingBox._max.z - boundingBox._min.z, &BoxMesh, 0);
|
绘制包围盒
绘制模型
D3DMATERIAL9 white = white_mtrl; white.Diffuse.a = 0.50f;
g_pd3dDevice->SetMaterial(&white);
if (boundingBox.isPointInside(D3DXVECTOR3(1, 1, 1))) { g_pd3dDevice->SetRenderState(D3DRS_FILLMODE, D3DFILL_WIREFRAME); BoxMesh->DrawSubset(0); g_pd3dDevice->SetRenderState(D3DRS_FILLMODE, D3DFILL_SOLID); }
|
效果
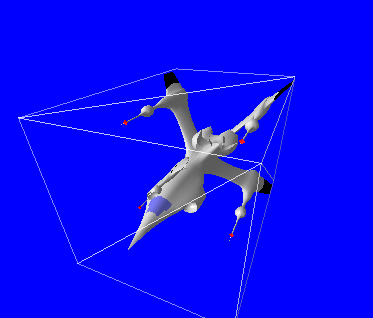